Plot Pixel Data: 2D Histogram Time Slice¶
Demonstration of how to plot a 2D histogram using only data from a specified time slice.
Luna Command¶
./tpx3dump process -i /Users/Ciaran/atlassian-bitbucket-pipelines-runner/temp/e71169e4-520a-5b30-a5ab-ee8a44eb5fac/build/docs/source/_static/example_data.tpx3 -o /Users/Ciaran/atlassian-bitbucket-pipelines-runner/temp/e71169e4-520a-5b30-a5ab-ee8a44eb5fac/build/docs/source/_static/example_data.h5 --eps-t 120ns --eps-s 2 --layout single
Python Script¶
Plot a 2D histogram of a time slice¶
1import os, sys
2import h5py # ensure you have `pip install h5py`
3import pandas as pd # ensure you have `pip install pandas`
4from typing import *
5import matplotlib.pyplot as plt
6
7import seaborn as sns
8sns.set_context(context="talk")
9
10# add some paths to PYTHONPATH
11for directory in ["..", "."]:
12 sys.path.append(os.path.abspath(os.path.join(os.path.dirname(__file__), directory)))
13
14# on our system "EXAMPLE_DATA_HDF5" refers to the absolute path
15# to a hdf5 file generated by luna. Replace with your own!
16from env_vars_for_docs_examples import EXAMPLE_DATA_HDF5, PLOTS_DIRECTORY
17
18# re-use function from previous 3_examples
19from ex2_read_data_time_units import load_pixel_hits, TimeUnit, convert_time_units
20
21
22
23def plot_2d_histogram_pixel_data(pixel_data: pd.DataFrame, variable: str, start_toa: float, toa_window_size: float):
24 """
25 Plots a 2D histogram of pixel data within an optional time window.
26
27 Args:
28 pixel_data (pd.DataFrame): DataFrame containing pixel data.
29 variable (str): The name of the variable to bin and plot
30 start_toa (float): The starting 'toa' value to filter data in the same units as pixel data
31 toa_window_size (float): The time window size to filter data.
32 """
33
34 end_toa = start_toa + toa_window_size
35 pixel_data = pixel_data[(pixel_data['toa'] >= start_toa) & (pixel_data['toa'] <= end_toa)]
36
37 fig, ax = plt.subplots(figsize=(10, 8))
38 h = ax.hist2d(pixel_data['x'], pixel_data['y'], weights=pixel_data[variable],
39 bins=[256, 256], range=[[0, 256], [0, 256]], cmap='BuPu')
40 plt.colorbar(h[3], ax=ax, label=f'Intensity of {variable}')
41 plt.title(f'2D Histogram of {variable} values by Pixel Coordinates')
42 plt.xlabel('Pixel X Coordinate')
43 plt.ylabel('Pixel Y Coordinate')
44 fname = os.path.join(PLOTS_DIRECTORY, f"ex4_plot_pixel_2d_histogram_tot_time_slice.png")
45 plt.savefig(fname, bbox_inches='tight', dpi=300)
46
47
48if __name__ == "__main__":
49
50 toa_units = TimeUnit.Seconds
51 pixel_hits: pd.DataFrame = load_pixel_hits(EXAMPLE_DATA_HDF5, toa_unit=toa_units)
52
53 start_time = pixel_hits.iloc[50]["toa"]
54
55 # 1 seconds worth of data
56 plot_2d_histogram_pixel_data(
57 pixel_data=pixel_hits, variable="tot",
58 start_toa=start_time, toa_window_size=1
59 )
Script Output¶
Example Output¶
hdf5 datasets: ['Clusters', 'ExposureTimeBoundaries', 'PixelHits']
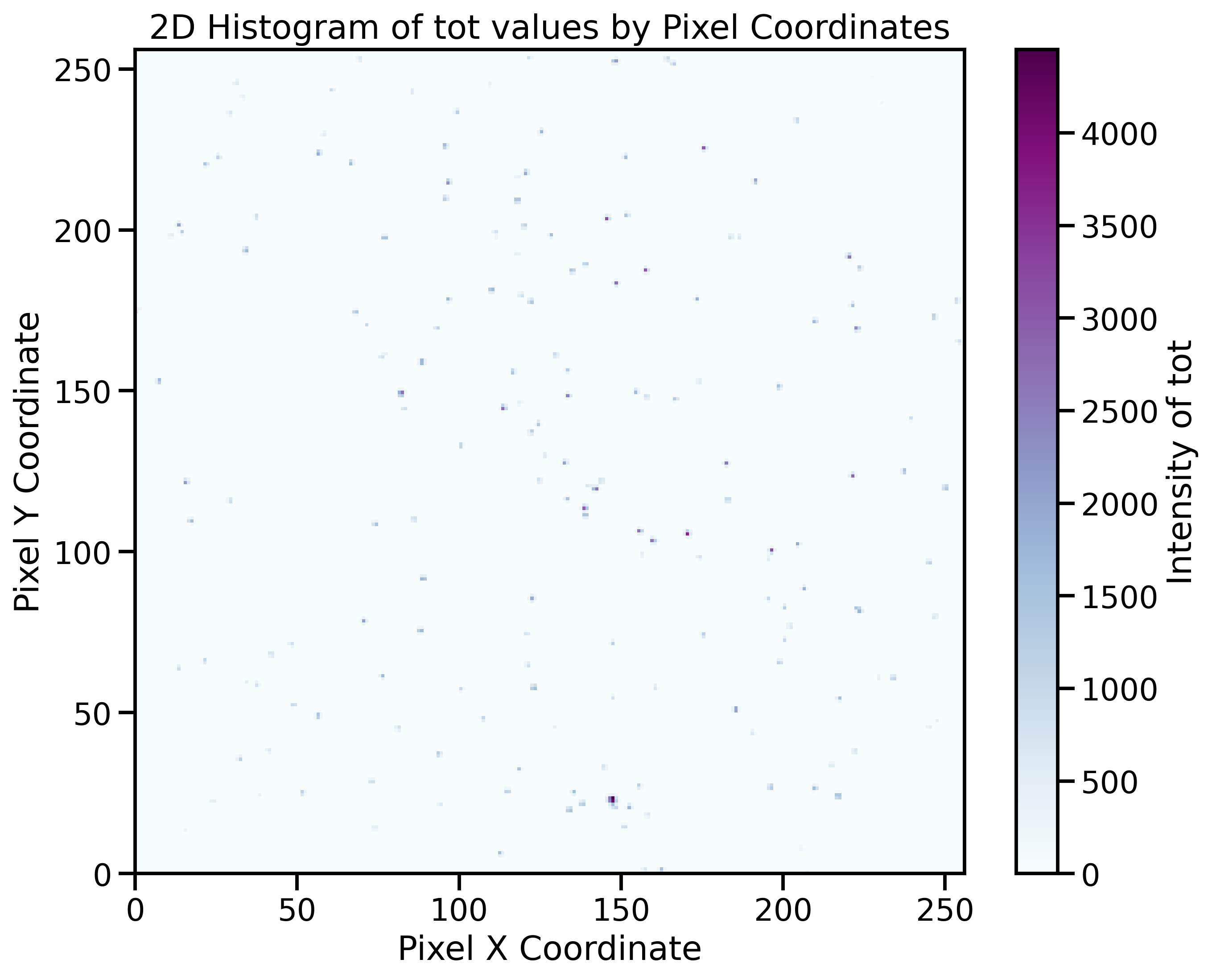