Plot Pixel Data: 2D Histogram¶
This example demonstrates how to read the HDF5 output of Luna using h5py and pandas and shows you how to convert the ToA time units to whatever you like.
Luna Command¶
./tpx3dump process -i /Users/Ciaran/atlassian-bitbucket-pipelines-runner/temp/e71169e4-520a-5b30-a5ab-ee8a44eb5fac/build/docs/source/_static/example_data.tpx3 -o /Users/Ciaran/atlassian-bitbucket-pipelines-runner/temp/e71169e4-520a-5b30-a5ab-ee8a44eb5fac/build/docs/source/_static/example_data.h5 --eps-t 100ns --eps-s 1 --layout single
Python Script¶
Plot a 2D histogram of pixel hits using matplotlib¶
1import os, sys
2import h5py # ensure you have `pip install h5py`
3import pandas as pd # ensure you have `pip install pandas`
4from typing import *
5import matplotlib.pyplot as plt
6
7import seaborn as sns
8sns.set_context(context="talk")
9
10# add some paths to PYTHONPATH
11for directory in ["..", "."]:
12 sys.path.append(os.path.abspath(os.path.join(os.path.dirname(__file__), directory)))
13
14# on our system "EXAMPLE_DATA_HDF5" refers to the absolute path
15# to a hdf5 file generated by luna. Replace with your own!
16from env_vars_for_docs_examples import EXAMPLE_DATA_HDF5, PLOTS_DIRECTORY
17
18# re-use pixel data loading function from example
19from ex2_read_data_time_units import load_pixel_hits, TimeUnit
20
21
22def plot_2d_histogram_pixel_data(pixel_data: pd.DataFrame, variable: str):
23 """
24 Plots a 2D histogram of pixel data.
25
26 The function plots a 2D histogram with pixel X and Y coordinates as axes and bins the values of the specified variable.
27
28 Args:
29 pixel_data (pd.DataFrame): DataFrame containing pixel data.
30 variable (str): The name of the variable to bin and plot.
31 """
32
33 fname = os.path.join(PLOTS_DIRECTORY, f"ex3_plot_pixel_2d_histogram_{variable}.png")
34
35 fig, ax = plt.subplots(figsize=(10, 8))
36
37 # Extracting x, y, and tot values
38 x = pixel_data['x']
39 y = pixel_data['y']
40 var = pixel_data[variable]
41
42 # Creating a 2D histogram
43 h = ax.hist2d(x, y, weights=var, bins=[256, 256], range=[[0, 256], [0, 256]], cmap='BuPu')
44
45 # Adding a colorbar
46 cbar = plt.colorbar(h[3], ax=ax)
47 cbar.set_label(f'Intensity of {variable}')
48
49 plt.title(f'2D Histogram of {variable} values by Pixel Coordinates')
50 plt.xlabel('Pixel X Coordinate')
51 plt.ylabel('Pixel Y Coordinate')
52
53 # Save the figure
54 plt.savefig(fname, bbox_inches='tight', dpi=300)
55
56
57if __name__ == "__main__":
58
59 pixel_hits: pd.DataFrame = load_pixel_hits(EXAMPLE_DATA_HDF5, toa_unit=TimeUnit.Microseconds)
60
61 plot_2d_histogram_pixel_data(pixel_data=pixel_hits, variable="tot")
Script Output¶
Example Output¶
hdf5 datasets: ['Clusters', 'ExposureTimeBoundaries', 'PixelHits']
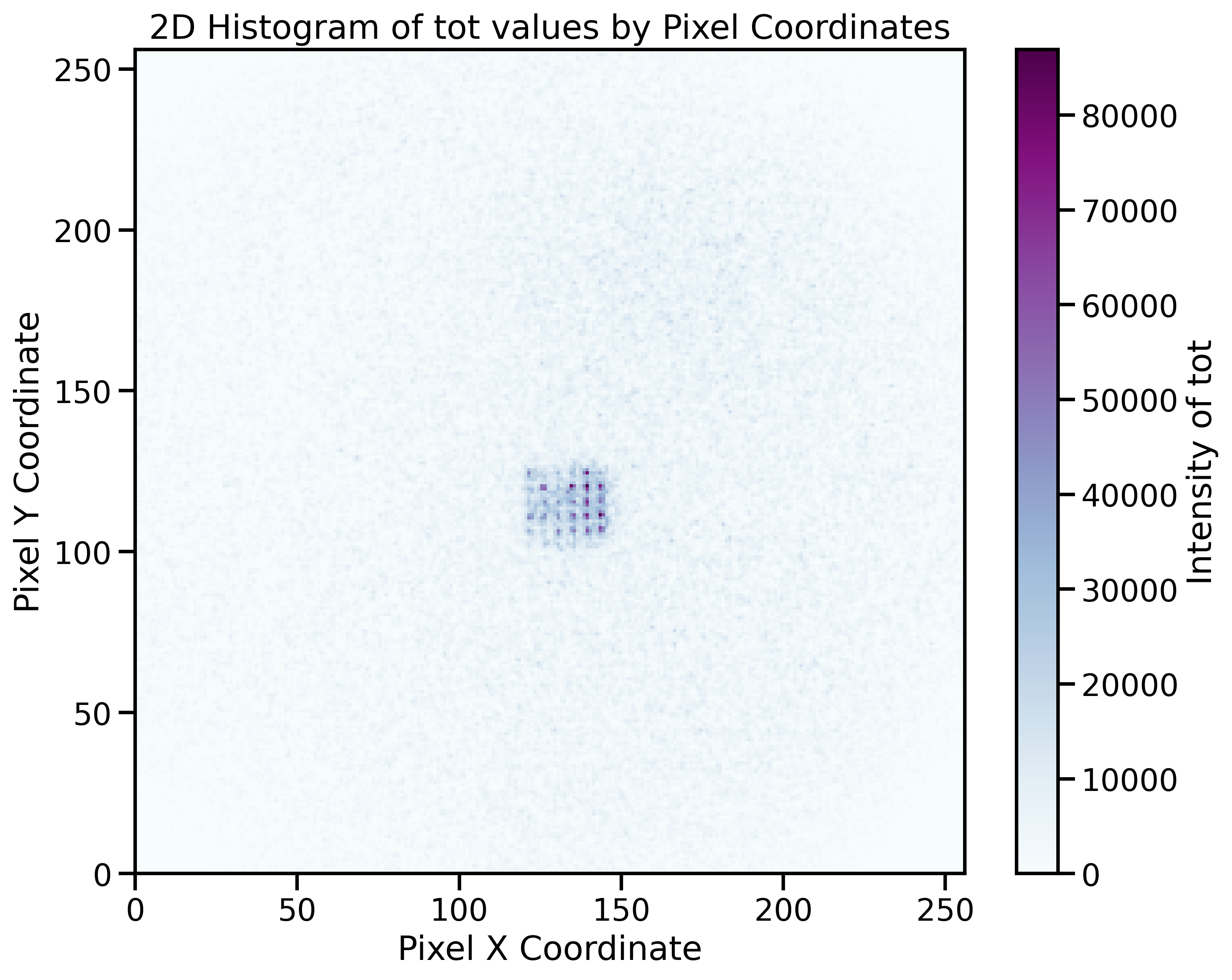